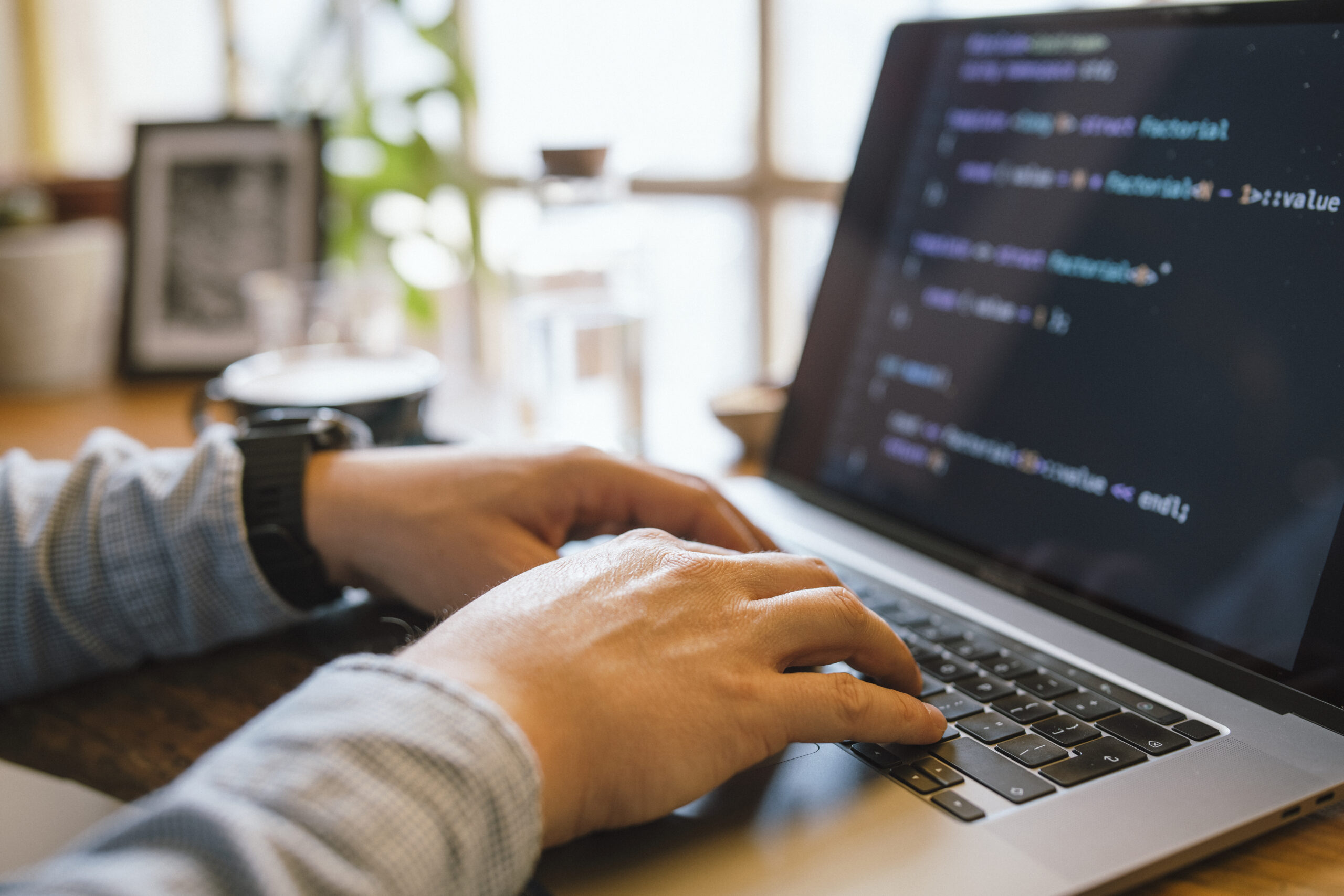
Debugging is One of the more important — nevertheless generally missed — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and Studying to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can conserve hours of aggravation and significantly enhance your productivity. Here are several procedures that will help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
On the list of fastest approaches developers can elevate their debugging skills is by mastering the applications they use on a daily basis. When composing code is 1 part of enhancement, figuring out the way to interact with it effectively through execution is equally important. Modern-day growth environments arrive Geared up with strong debugging capabilities — but many builders only scratch the surface of what these applications can perform.
Consider, for example, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These tools assist you to set breakpoints, inspect the worth of variables at runtime, phase via code line by line, and perhaps modify code within the fly. When used effectively, they Allow you to notice specifically how your code behaves during execution, that's a must have for monitoring down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-close developers. They help you inspect the DOM, check community requests, check out serious-time functionality metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can flip discouraging UI concerns into workable responsibilities.
For backend or technique-amount developers, applications like GDB (GNU Debugger), Valgrind, or LLDB present deep Manage in excess of functioning procedures and memory administration. Understanding these instruments may have a steeper Finding out curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn into snug with Edition Management systems like Git to grasp code record, discover the exact instant bugs were launched, and isolate problematic variations.
Ultimately, mastering your equipment usually means likely beyond default settings and shortcuts — it’s about establishing an personal knowledge of your improvement surroundings to make sure that when problems come up, you’re not missing at the hours of darkness. The greater you are aware of your tools, the more time you'll be able to expend resolving the particular issue as opposed to fumbling by means of the method.
Reproduce the challenge
One of the more critical — and often disregarded — measures in productive debugging is reproducing the issue. Before leaping into your code or earning guesses, builders will need to produce a reliable natural environment or state of affairs where by the bug reliably seems. With no reproducibility, repairing a bug gets a match of likelihood, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a dilemma is collecting just as much context as is possible. Request questions like: What steps resulted in The problem? Which surroundings was it in — improvement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact disorders beneath which the bug occurs.
As you’ve collected enough information and facts, endeavor to recreate the trouble in your neighborhood setting. This could signify inputting the identical details, simulating equivalent person interactions, or mimicking program states. If The difficulty appears intermittently, look at creating automatic tests that replicate the edge conditions or state transitions included. These tests not simply help expose the challenge but will also prevent regressions Sooner or later.
In some cases, the issue can be surroundings-precise — it might come about only on sure working programs, browsers, or less than specific configurations. Making use of instruments like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a frame of mind. It involves persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you are presently halfway to repairing it. By using a reproducible circumstance, You should utilize your debugging applications extra effectively, test potential fixes safely, and communicate more clearly with your team or users. It turns an summary criticism right into a concrete problem — and that’s in which developers thrive.
Study and Understand the Mistake Messages
Mistake messages in many cases are the most worthy clues a developer has when anything goes Mistaken. As an alternative to viewing them as irritating interruptions, developers should really master to deal with error messages as immediate communications with the process. They typically let you know exactly what transpired, wherever it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Commence by reading the information meticulously and in comprehensive. Quite a few developers, specially when underneath time stress, glance at the 1st line and right away start building assumptions. But deeper in the mistake stack or logs may lie the genuine root result in. Don’t just copy and paste mistake messages into engines like google — read and have an understanding of them very first.
Crack the mistake down into elements. Can it be a syntax error, a runtime exception, or simply a logic error? Will it stage to a selected file and line amount? What module or functionality induced it? These issues can manual your investigation and place you toward the dependable code.
It’s also helpful to grasp the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java frequently comply with predictable styles, and Understanding to acknowledge these can drastically accelerate your debugging process.
Some problems are imprecise or generic, and in Individuals scenarios, it’s crucial to examine the context where the error occurred. Check out similar log entries, input values, and recent alterations during the codebase.
Don’t neglect compiler or linter warnings both. These typically precede larger sized issues and provide hints about likely bugs.
Finally, mistake messages are not your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is One of the more powerful tools inside a developer’s debugging toolkit. When employed efficiently, it provides actual-time insights into how an application behaves, aiding you realize what’s taking place beneath the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with understanding what to log and at what level. Common logging levels include DEBUG, Facts, Alert, Mistake, and Deadly. Use DEBUG for specific diagnostic data for the duration of growth, Information for common events (like successful get started-ups), Alert for prospective problems that don’t crack the applying, ERROR for actual problems, and Lethal if the process can’t keep on.
Stay away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure significant messages and slow down your system. Center on essential activities, point out alterations, input/output values, and important selection details with your code.
Format your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and function names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Enable you to track how variables evolve, what ailments are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re In particular beneficial in generation environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about equilibrium and clarity. Using a very well-thought-out logging technique, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and Increase the All round maintainability and dependability within your code.
Think Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly establish and fix bugs, developers should technique the method similar to a detective resolving a secret. This attitude can help stop working elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or performance issues. Much like a detective surveys a crime scene, collect as much relevant information as you are able to with out jumping to conclusions. Use logs, test cases, and person experiences to piece jointly a transparent image of what’s taking place.
Subsequent, form hypotheses. Ask yourself: What can be producing this habits? Have any alterations just lately been created towards the codebase? Has this issue happened before below comparable instances? The target will be to slender down alternatives and determine opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the trouble inside a managed ecosystem. For those who suspect a selected perform or component, isolate it and validate if The problem persists. Similar to a detective conducting interviews, check with your code issues and Allow the outcomes guide you nearer to the reality.
Pay out shut consideration to tiny details. Bugs normally cover within the the very least expected destinations—just like a missing semicolon, an off-by-1 mistake, or perhaps a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without entirely comprehending it. Momentary fixes might cover the real dilemma, just for it to resurface later.
And lastly, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future concerns and assistance Other individuals fully grasp your reasoning.
By considering just like a detective, builders can sharpen their analytical competencies, method challenges methodically, and become more effective at uncovering hidden difficulties in complex techniques.
Produce Checks
Writing tests is one of the best solutions to help your debugging abilities and All round growth performance. Checks don't just help catch bugs early but additionally serve as a safety net that provides you self confidence when building variations towards your codebase. A well-tested software Gustavo Woltmann AI is much easier to debug because it allows you to pinpoint precisely exactly where and when an issue occurs.
Start with unit checks, which deal with unique capabilities or modules. These compact, isolated checks can promptly expose no matter if a certain bit of logic is Doing work as anticipated. Whenever a check fails, you instantly know where to glimpse, noticeably cutting down enough time put in debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear soon after previously being preset.
Following, integrate integration checks and conclusion-to-conclude exams into your workflow. These help make sure a variety of elements of your software get the job done collectively smoothly. They’re specially helpful for catching bugs that manifest in intricate methods with various parts or solutions interacting. If a little something breaks, your exams can show you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to Consider critically about your code. To test a feature appropriately, you'll need to be aware of its inputs, expected outputs, and edge scenarios. This degree of knowledge Normally potential customers to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. When the exam fails constantly, you may concentrate on repairing the bug and check out your test move when The difficulty is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing match right into a structured and predictable process—aiding you catch additional bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to be immersed in the issue—looking at your display for hrs, striving Option just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen stress, and sometimes see The problem from a new viewpoint.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You might start overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain gets to be much less efficient at problem-resolving. A brief walk, a coffee crack, or maybe switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report discovering the foundation of a challenge once they've taken time for you to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also help reduce burnout, In particular in the course of lengthier debugging classes. Sitting in front of a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Strength along with a clearer mindset. You would possibly quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you before.
When you’re stuck, a very good guideline should be to set a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that point to move all around, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily beneath limited deadlines, however it essentially results in speedier and more effective debugging Eventually.
To put it briefly, using breaks will not be a sign of weak point—it’s a wise strategy. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you experience is much more than simply A short lived setback—it's a chance to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can instruct you some thing useful in case you go to the trouble to replicate and analyze what went Incorrect.
Commence by asking by yourself a number of critical thoughts as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code critiques, or logging? The answers frequently reveal blind places in your workflow or understanding and help you build stronger coding patterns going ahead.
Documenting bugs can even be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or prevalent problems—which you could proactively stay away from.
In group environments, sharing what you've figured out from a bug together with your friends is often Specially potent. Whether it’s via a Slack concept, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your mindset from irritation to curiosity. As opposed to dreading bugs, you’ll start appreciating them as vital elements of your progress journey. In any case, a lot of the ideal builders usually are not those who create great code, but those that repeatedly discover from their faults.
In the end, Every single bug you fix adds a different layer for your ability established. So subsequent time you squash a bug, take a instant to reflect—you’ll arrive absent a smarter, more capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It would make you a far more effective, assured, and able developer. Another time you're knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.